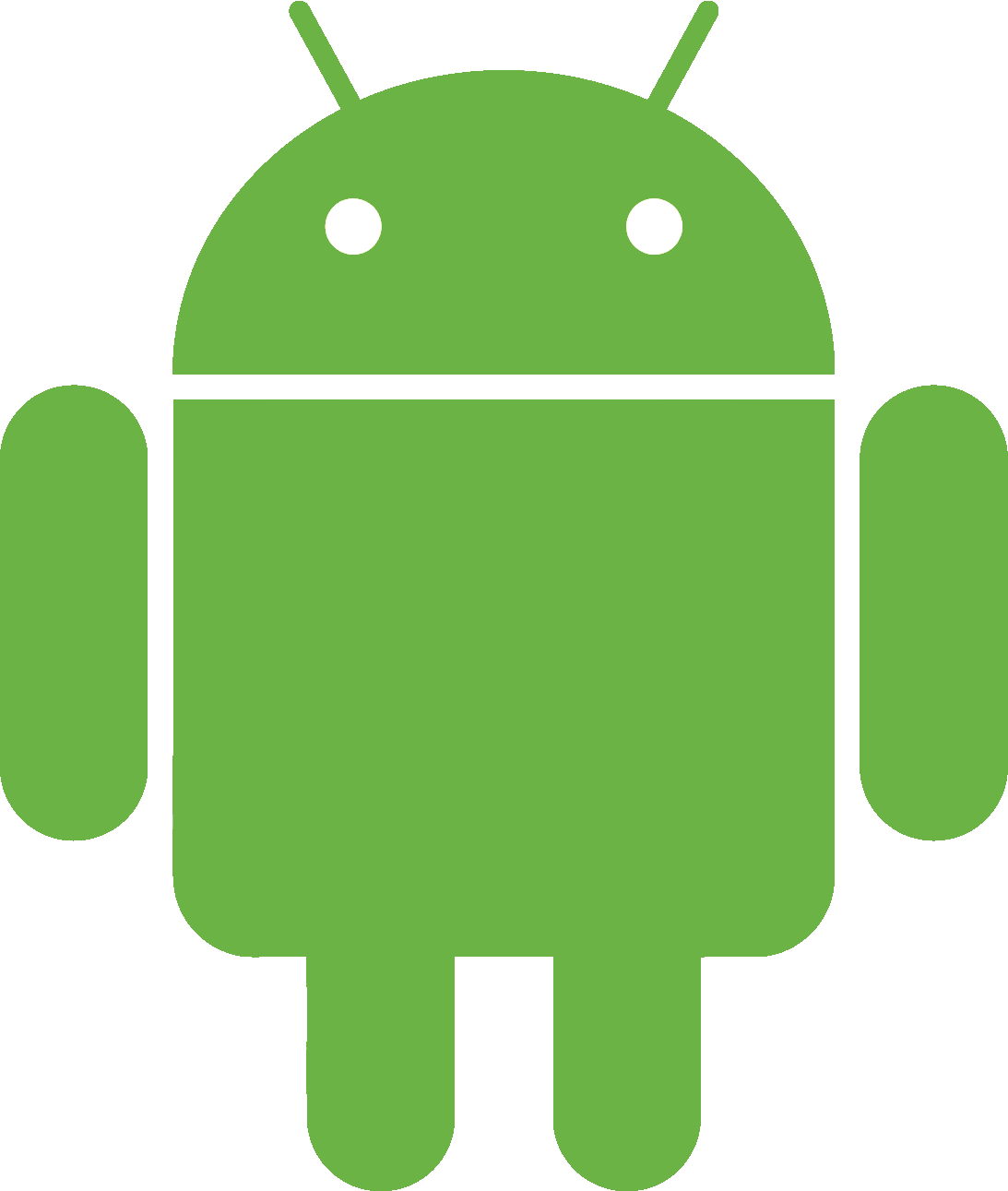
* Most of the material is taken from these youtube videos.
Official Documentation can be found here.
Keywords and Concepts
- Views are kind of the generic term for any item we put into our apps layout.
- Activity means a page in the app.
- Intents are for activities talking to each other.
- R refers to resource class
- Broadcasts send systemwide messages and can be used for communication between different apps.
- Threads enable parallel execution of code in contrast to the default sequential execution (e.x. if there is long calculations which causes the app to hang)
- Services are chunks of code that run in the background (e.x. social network app that checks for notifications, or for a download). Services don't have user interface.
Options
- layout:weight sets what proportion of the screen the layout gets (between 0 and 1)
- id is used for refering to things in the code layer
Databases
- Each row in a database file need to be represented by an object in java
Misc.
- Use dp or sp for size unit.
- Put images in drawable folder.
- Disable "Code Folding" and add "Auto Import" in the preferences.
- To delete action bar (the bar at the top by default) change styles.xml in values folder (.NoActionBar)
Examples
When button "about" (id) is clicked, show the page "aboutPage"
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button aboutBtn = (Button) findViewById(R.id.about);
aboutBtn.setOnClickListener(new View.OnClickListener() { //event handling
@Override
public void onClick(View v) {
gotoAbout(); //CallBack method
}
});
}
private void gotoAbout() {
Intent intent = new Intent(this, aboutPage.class);
startActivity(intent);
}
}
An easier approach is to add android:onClick="clickBtn" to the button attributes in the xml file, and define the clickBtn in java code as follows:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void clickBtn(View view) {
Intent intent = new Intent(this, aboutPage.class);
startActivity(intent);
}
}
To pass information along with transition to the new page: (suppose we have a text field (id: textField) in the main page where user enters his name. when he presses the button we want to go to about page and show his name in a TextView element (id: aboutPageText))
We need to extend clickBtn as follows
public void clickBtn(View view) {
Intent intent = new Intent(this, aboutPage.class);
final EditText textField1 = (EditText) findViewById(R.id.textField); //referrence to input field
String userMsg = textField1.getText().toString();
intent.putExtra("Msg", userMsg);
startActivity(intent);
}
and add the following to aboutPage.java in the onCreate method:
Bundle comingInfo = getIntent().getExtras();
if (comingInfo==null) {
return;
}
String MsgContent = comingInfo.getString("Msg");
final TextView newPageText = (TextView) findViewById(R.id.aboutPageText);
newPageText.setText(MsgContent);